Effective Java
Duration (in days):
4
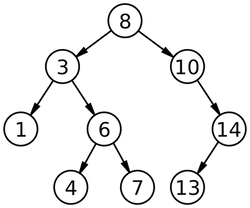
Description:
Mastery of Java is not easy. In this course, we have collected best practices and patterns to help Java programmers move from fluency to mastery.
We cover many of the best practices from Joshua Bloch book, but in addition, we have added examples of patterns and anti-patterns that we and our customers have experienced.
Objectives:
Learn how to write effective Java programs
Avoid common costly traps
Prerequisites:
Mastery of Java is not easy. In this course, we have collected best practices and patterns to help Java programmers move from fluency to mastery.
We cover many of the best practices from Joshua Bloch book, but in addition, we have added examples of patterns and anti-patterns that we and our customers have experienced.
Audience
Java programmers
Outline
Creating and destroying objects
Static factory methods
Builders
Singletons
How to avoid creating unnecessary objects?
Common object methods
Proper use of equals
Proper use of hashCode
Proper implementation of toString
Cloning objects
Mastering classes and interfaces
Accesibility of members and classes
Accessors vs. fields
Composition vs. inheritance
Proper use of Interfaces
Proper design of class hierarchies
Implementation strategies with function objects
Generics
Avoiding raw types
Facvoring generic colections
Creating generic types and metods
The rule of PECS
Increasing API flexibility
Enumeration
Defining enumeration
Using annotations
Proper use of enumeration and annotations
Mastering methods
Designing method signatures
Validate paramters
Method overloading
Overloading, generics, and autoboxing
Default methods
Using variable lists of arguments
Programming techniques
For-each loops
Minimizing scope of local variables
Working with exact numbers
Strings vs. more meaningful classes
Interfaces vs. reflection
Working with exceptions
Handling exceptional conditions
Using standard exceptions
Unnecessary checked exceptions
Exceptions and abstractions
Capture failure information
Java NIO
High-speed I/O
Buffers
Channels
Schedulers
Proper use of NIO
java.util.concurrent
Executors
Futures
BlockingQueue
CountdownLatch
Locks
Semaphores
Exchanges
Atomic variables
Callable
Java Lambda
Lambda syntax
Why Lambda?
Closures
Function composition
Monoids
Monads
Java Streaming
Essential concepts
Streaming functions
Parallelism and streaming
Streaming alternatives