Node.js
Duration (in days):
3
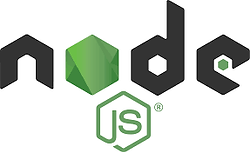
Description:
Node.js has become a very popular framwork to build the underlying logic of an application. In this course, we'll give the students a solid understanding of Node.js, it's infrastucture, the supporting tools.
Objectives:
Master the node.js develoment environment
Learn how to effectively debug node.js applications
Learn how to package your node.js componentws
Learn how to build a web-site using node.js
Learn some of the more popular frameworks for node.js
Prerequisites:
Node.js has become a very popular framwork to build the underlying logic of an application. In this course, we'll give the students a solid understanding of Node.js, it's infrastucture, the supporting tools.
Audience
JavaScript programmers
Outline
Introduction to Node.js
What is Node.js?
History of Node.js
Why is Node.js important?
Industry use cases and the result of applying Node.js in known enterprises
How does Node.js work?
Other asynchronous frameworks
Node.js components
Node Core
Userland
What do we mean by event-driven?
Blocking vs. non-blocking I/O
V8 and JavaScript
The EchoServer
Asychronous programming techniques
Synchronous vs. asynchronous programming
ES6
JavaScript closures
Callback pattern
Event emitters and listeners
Exceptions and error handling
Managing identities in callbacks
Flow control
Deferring function execution
Controlling the Node event loop
Modules
What is a Module?
Understanding the anatomy of a module
Package management
NPM and node_modules
Semver
Writing your own module
How to debug Node.js?
Embedded debugging code
Node.js' debugging client
Debugging with IDEs
Chrome Developer Tools
Node Debugging Guide
Best practices
Test-driven development in Node.js
Unit testing in Node
Assert module
Nodeunit
Mocha
Other testing frameworks
Accessing the file system
File system module fs
File statistics
Watching file modifications
Reading files
Writing to files
Streams and buffers
Reading
Writing
Pipes
Chaining
Handling binary data
Networking
The networking module net
Creating servers
Creating networking clients
Building web servers
Node.js Hello World Server
Servers
Responses
Requests
URLs
Query Strings
Clients
Security with HTTPS
Express
What is Expess?
Middleware
Error handling
Generating an application skeleton
Configuration
Routing
Handling downloads and uploads
Authentication
Express middleware
Cookies
Body parsing
Logging
Sessions
Method override
Basic webserver functionality
VHost
Express-session
CSRF
Compression
Serve-favicon
Serve-static
Serve-index
Restful express
What is REST?
REST and express
Example of a REST API
REST vs GraphQL
Async
The boomerang effect
async.series
async.parallel
async.waterfall
async.auto
async.each
async.map
Recommendations and best practices
Async alternatives
Promises
What is a promise?
Creating a promise
Promises API
Promises and errors
Parallel
Async / await
Best practices